Warning: mysqli_query(): (HY000/3): Error writing file '/tmp/MYBsifvX' (Errcode: 28 - No space left on device) in /www/wwwroot/www.ksyll.com/wp-includes/class-wpdb.php on line 2351
今天公司给安排了一个任务,想着以后可能用到将文件导出为pdf的情况挺多的,让我做一个导出pdf的类库。于是乎就有了我下面写的代码,记录分享一下!(ps:我注释一般写的很多)
using iTextSharp.text;
using iTextSharp.text.pdf;
using System.Collections.Generic;
using System.Diagnostics;
using System.IO;
namespace ToPdfHelper
{
/// <summary>
/// 导出为PDF
/// </summary>
/// <typeparam name="T">实体类型</typeparam>
public static class PDFExporter<T>
{
/// <summary>
/// 导出数据到PDF
/// </summary>
/// <param name="filePath">导出的文件Or路径名</param>
/// <param name="data">需要导出的数据</param>
/// <param name="columnWidths">每列的宽度</param>
/// <param name="headerTitles">表头</param>
/// <param name="title">首行插入的标题</param>
/// <param name="pageSize">纸张类型Or大小</param>
/// <param name="fontPath">设置字体路径</param>
public static void ExportDataToPDF(string filePath, List<T> data, float[] columnWidths, string[] headerTitles, string title, Rectangle pageSize, string fontPath)
{
// 创建文件流
using (FileStream fs = new FileStream(filePath, FileMode.Create))
{
// 创建文档对象
Document doc = new Document(pageSize);
// 创建PDF写入器
PdfWriter writer = PdfWriter.GetInstance(doc, fs);
// 打开文档
doc.Open();
// 创建字体
BaseFont baseFont = BaseFont.CreateFont(fontPath, BaseFont.IDENTITY_H, BaseFont.NOT_EMBEDDED);
Font font = new Font(baseFont, 14f, Font.BOLD);
// 添加标题
if (!string.IsNullOrEmpty(title))
{
Paragraph titleParagraph = new Paragraph(title, font);
titleParagraph.Alignment = Element.ALIGN_CENTER;
doc.Add(titleParagraph);
doc.Add(new Paragraph("n"));
}
// 创建表格对象
PdfPTable table = new PdfPTable(columnWidths.Length);
// 设置表格列宽
table.SetWidths(columnWidths);
// 添加表头
foreach (string headerTitle in headerTitles)
{
table.AddCell(new Phrase(headerTitle, font));
}
// 添加表格数据
foreach (T rowData in data)
{
foreach (var propertyInfo in typeof(T).GetProperties())
{
table.AddCell(new Phrase(propertyInfo.GetValue(rowData)?.ToString(), font));
}
}
// 将表格添加到文档中
doc.Add(table);
// 关闭文档
doc.Close();
// 打开 PDF 文档
Process.Start(filePath);
}
}
}
}
调用示例:
图方便,就用控制台调用了,别打我真不是我懒
using iTextSharp.text;
using System.Collections.Generic;
using ToPdfHelper;
namespace PdfTest
{
internal class Program
{
static void Main(string[] args)
{
List<Employee> data = new List<Employee>
{
new Employee() { Name = "John", Age = 25, Gender = "Male" },
new Employee() { Name = "Jane", Age = 30, Gender = "Female" }
};
// 创建中文字体
string fontPath = "C:\Windows\Fonts\msyh.ttc,0";
float[] columnWidths = new float[] { 2f, 1f, 1f };
string[] headerTitle = { "名字", "年龄", "姓氏" };
string title = "Employee Information";
Rectangle pageSize = PageSize.A4;
PDFExporter<Employee>.ExportDataToPDF(@"D:test.pdf", data, columnWidths, headerTitle, title, pageSize, fontPath);
}
public class Employee
{
public string Name { get; set; }
public int Age { get; set; }
public string Gender { get; set; }
}
}
}
效果图:
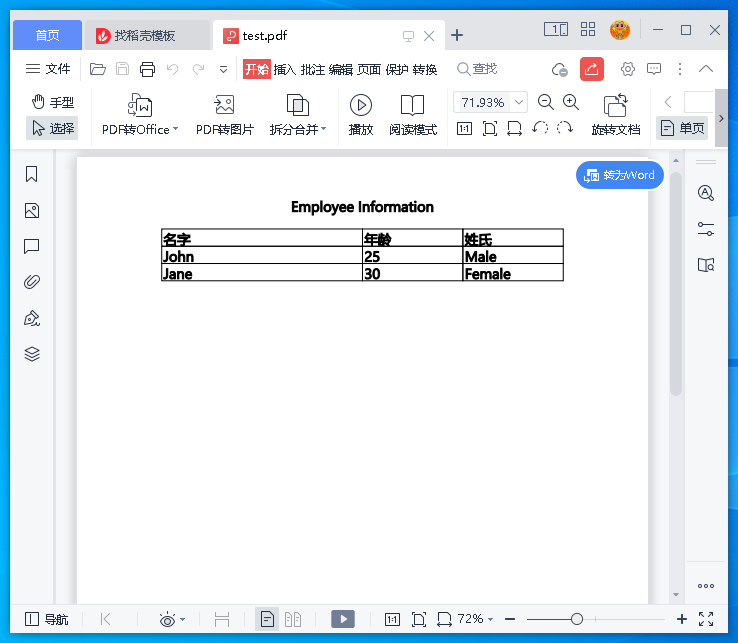
相关推荐: LINQ之删除属性值为null或者为空字符串的字段 今天碰到一个要求,需要底物瓶号码,SubstrateBottleNo参数 1 或 2. 仅仅当上述PipeLineNo参数值为7/8时候此参数有效。我做了处理之后,发现SubstrateBottleNo没有给它赋值之后,页面显示了一个空的,这时候需要把空的值删…
© 版权声明
THE END
暂无评论内容